mirror of
https://github.com/kevinporetti/UnrealImGui.git
synced 2025-01-18 16:30:32 +00:00
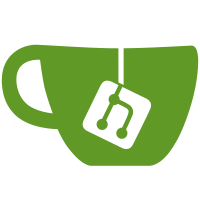
- The old DPI Scale setting was replaced by DPI Scaling Info, which contains information about scale and method of scaling. - ImGui Context Manager handles scaling in ImGui by scaling all styles, rebuilding fonts using a different size, and raising OnFontAtlasBuilt event. - ImGui Module Manager uses OnFontAtlasBuilt event to rebuild font textures. - The update policy in Texture Manager was loosened to update existing resources rather than throwing an exception. This is less strict but it is now more useful since our main texture can now change. The throwing behavior used in the public interface is now handled before calling to the Texture Manager.
97 lines
2.7 KiB
C++
97 lines
2.7 KiB
C++
// Distributed under the MIT License (MIT) (see accompanying LICENSE file)
|
|
|
|
#pragma once
|
|
|
|
#include "ImGuiContextManager.h"
|
|
#include "ImGuiDemo.h"
|
|
#include "ImGuiModuleCommands.h"
|
|
#include "ImGuiModuleProperties.h"
|
|
#include "ImGuiModuleSettings.h"
|
|
#include "TextureManager.h"
|
|
#include "Widgets/SImGuiLayout.h"
|
|
|
|
|
|
// Central manager that implements module logic. It initializes and controls remaining module components.
|
|
class FImGuiModuleManager
|
|
{
|
|
// Allow module to control life-cycle of this class.
|
|
friend class FImGuiModule;
|
|
|
|
public:
|
|
|
|
// Get interface to module settings.
|
|
FImGuiModuleSettings& GetSettings() { return Settings; }
|
|
|
|
// Get interface to module state properties.
|
|
FImGuiModuleProperties& GetProperties() { return Properties; }
|
|
|
|
// Get ImGui contexts manager.
|
|
FImGuiContextManager& GetContextManager() { return ContextManager; }
|
|
|
|
// Get texture resources manager.
|
|
FTextureManager& GetTextureManager() { return TextureManager; }
|
|
|
|
// Event called right after ImGui is updated, to give other subsystems chance to react.
|
|
FSimpleMulticastDelegate& OnPostImGuiUpdate() { return PostImGuiUpdateEvent; }
|
|
|
|
private:
|
|
|
|
FImGuiModuleManager();
|
|
~FImGuiModuleManager();
|
|
|
|
FImGuiModuleManager(const FImGuiModuleManager&) = delete;
|
|
FImGuiModuleManager& operator=(const FImGuiModuleManager&) = delete;
|
|
|
|
FImGuiModuleManager(FImGuiModuleManager&&) = delete;
|
|
FImGuiModuleManager& operator=(FImGuiModuleManager&&) = delete;
|
|
|
|
void LoadTextures();
|
|
void BuildFontAtlasTexture();
|
|
|
|
bool IsTickRegistered() { return TickDelegateHandle.IsValid(); }
|
|
void RegisterTick();
|
|
void UnregisterTick();
|
|
|
|
void CreateTickInitializer();
|
|
void ReleaseTickInitializer();
|
|
|
|
void Tick(float DeltaSeconds);
|
|
|
|
void OnViewportCreated();
|
|
|
|
void AddWidgetToViewport(UGameViewportClient* GameViewport);
|
|
void AddWidgetsToActiveViewports();
|
|
|
|
void OnContextProxyCreated(int32 ContextIndex, FImGuiContextProxy& ContextProxy);
|
|
|
|
// Event that we call after ImGui is updated.
|
|
FSimpleMulticastDelegate PostImGuiUpdateEvent;
|
|
|
|
// Collection of module state properties.
|
|
FImGuiModuleProperties Properties;
|
|
|
|
// Tying module console commands to life-cycle of this manager and module.
|
|
FImGuiModuleCommands Commands;
|
|
|
|
// ImGui settings proxy (valid in every loading stage).
|
|
FImGuiModuleSettings Settings;
|
|
|
|
// Widget that we add to all created contexts to draw ImGui demo.
|
|
FImGuiDemo ImGuiDemo;
|
|
|
|
// Manager for ImGui contexts.
|
|
FImGuiContextManager ContextManager;
|
|
|
|
// Manager for textures resources.
|
|
FTextureManager TextureManager;
|
|
|
|
// Slate widgets that we created.
|
|
TArray<TWeakPtr<SImGuiLayout>> Widgets;
|
|
|
|
FDelegateHandle TickInitializerHandle;
|
|
FDelegateHandle TickDelegateHandle;
|
|
FDelegateHandle ViewportCreatedHandle;
|
|
|
|
bool bTexturesLoaded = false;
|
|
};
|