mirror of
https://github.com/kevinporetti/UnrealImGui.git
synced 2025-07-09 22:00:33 +00:00
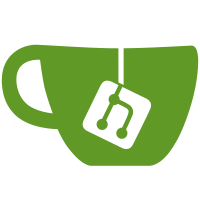
Using ImGui to draw cursor helps to reduce visual error between mouse cursor and position in ImGui. But it has been reported that this works really bad when frame-rate drops and becomes unusable below ~20 FPS. Taking that into account hardware cursor seems like a better default option. Old behaviour can be enabled with ImGui.DrawMouseCursor console variable.
100 lines
3.3 KiB
C++
100 lines
3.3 KiB
C++
// Distributed under the MIT License (MIT) (see accompanying LICENSE file)
|
|
|
|
#pragma once
|
|
|
|
#include "TextureManager.h"
|
|
|
|
#include <ICursor.h>
|
|
|
|
#include <imgui.h>
|
|
|
|
|
|
class FImGuiInputState;
|
|
|
|
// Utilities to help standardise operations between Unreal and ImGui.
|
|
namespace ImGuiInterops
|
|
{
|
|
//====================================================================================================
|
|
// ImGui Types
|
|
//====================================================================================================
|
|
|
|
namespace ImGuiTypes
|
|
{
|
|
using FMouseButtonsArray = decltype(ImGuiIO::MouseDown);
|
|
using FKeysArray = decltype(ImGuiIO::KeysDown);
|
|
|
|
using FInputCharactersBuffer = decltype(ImGuiIO::InputCharacters);
|
|
|
|
using FKeyMap = decltype(ImGuiIO::KeyMap);
|
|
}
|
|
|
|
|
|
//====================================================================================================
|
|
// Input Mapping
|
|
//====================================================================================================
|
|
|
|
// Set in ImGui IO mapping to recognize indices generated from Unreal input events.
|
|
void SetUnrealKeyMap(ImGuiIO& IO);
|
|
|
|
// Map FKey to index in keys buffer.
|
|
uint32 GetKeyIndex(const FKey& Key);
|
|
|
|
// Map key event to index in keys buffer.
|
|
FORCEINLINE uint32 GetKeyIndex(const FKeyEvent& KeyEvent)
|
|
{
|
|
return KeyEvent.GetKeyCode();
|
|
}
|
|
|
|
// Map mouse FKey to index in mouse buttons buffer.
|
|
uint32 GetMouseIndex(const FKey& MouseButton);
|
|
|
|
// Map pointer event to index in mouse buttons buffer.
|
|
FORCEINLINE uint32 GetMouseIndex(const FPointerEvent& MouseEvent)
|
|
{
|
|
return GetMouseIndex(MouseEvent.GetEffectingButton());
|
|
}
|
|
|
|
EMouseCursor::Type ToSlateMouseCursor(ImGuiMouseCursor MouseCursor);
|
|
|
|
|
|
//====================================================================================================
|
|
// Input State Copying
|
|
//====================================================================================================
|
|
|
|
// Copy input to ImGui IO.
|
|
// @param IO - Target ImGui IO
|
|
// @param InputState - Input state to copy
|
|
void CopyInput(ImGuiIO& IO, const FImGuiInputState& InputState);
|
|
|
|
|
|
//====================================================================================================
|
|
// Conversions
|
|
//====================================================================================================
|
|
|
|
// Convert from ImGui packed color to FColor.
|
|
FORCEINLINE FColor UnpackImU32Color(ImU32 Color)
|
|
{
|
|
// We use IM_COL32_R/G/B/A_SHIFT macros to support different ImGui configurations.
|
|
return FColor{ (uint8)((Color >> IM_COL32_R_SHIFT) & 0xFF), (uint8)((Color >> IM_COL32_G_SHIFT) & 0xFF),
|
|
(uint8)((Color >> IM_COL32_B_SHIFT) & 0xFF), (uint8)((Color >> IM_COL32_A_SHIFT) & 0xFF) };
|
|
}
|
|
|
|
// Convert from ImVec4 rectangle to FSlateRect.
|
|
FORCEINLINE FSlateRect ToSlateRect(const ImVec4& ImGuiRect)
|
|
{
|
|
return FSlateRect{ ImGuiRect.x, ImGuiRect.y, ImGuiRect.z, ImGuiRect.w };
|
|
}
|
|
|
|
// Convert from ImGui Texture Id to Texture Index that we use for texture resources.
|
|
FORCEINLINE TextureIndex ToTextureIndex(ImTextureID Index)
|
|
{
|
|
return static_cast<TextureIndex>(reinterpret_cast<intptr_t>(Index));
|
|
}
|
|
|
|
// Convert from Texture Index to ImGui Texture Id that we pass to ImGui.
|
|
FORCEINLINE ImTextureID ToImTextureID(TextureIndex Index)
|
|
{
|
|
return reinterpret_cast<ImTextureID>(static_cast<intptr_t>(Index));
|
|
}
|
|
}
|