mirror of
https://github.com/kevinporetti/UnrealImGui.git
synced 2025-01-18 16:30:32 +00:00
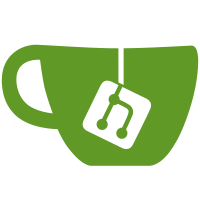
Note that this commit contains a minor breaking change for custom implementations of UImGuiInputHandler. - Changed "ImGui.SwitchInputMode" command name to "ImGui.ToggleInput". - Changed SwitchInputModeKey property in settings to ToggleInput. - Added temporary mechanism protecting from losing data by moving it from SwitchInputModeKey to ToggleInput and removing deprecated entry from the default config file. - Renamed UImGuiInputHandler protected function from IsSwitchInputModeEvent to IsToggleInputEvent, so custom implementations of that class may require update.
75 lines
2.0 KiB
C++
75 lines
2.0 KiB
C++
// Distributed under the MIT License (MIT) (see accompanying LICENSE file)
|
|
|
|
#include "ImGuiPrivatePCH.h"
|
|
|
|
#include "ImGuiModuleCommands.h"
|
|
|
|
#include "ImGuiSettings.h"
|
|
#include "Utilities/DebugExecBindings.h"
|
|
|
|
|
|
namespace CommandNames
|
|
{
|
|
namespace
|
|
{
|
|
const TCHAR* ToggleInput = TEXT("ImGui.ToggleInput");
|
|
}
|
|
}
|
|
|
|
FImGuiModuleCommands::FImGuiModuleCommands()
|
|
: ToggleInputCommand(CommandNames::ToggleInput,
|
|
TEXT("Switch ImGui input mode."),
|
|
FConsoleCommandDelegate::CreateRaw(this, &FImGuiModuleCommands::ToggleInput))
|
|
{
|
|
// Delegate initializer to support settings loaded after this object creation (in stand-alone builds) and potential
|
|
// reloading of settings.
|
|
UImGuiSettings::OnSettingsLoaded().AddRaw(this, &FImGuiModuleCommands::InitializeSettings);
|
|
|
|
// Call initializer to support settings already loaded (editor).
|
|
InitializeSettings();
|
|
}
|
|
|
|
FImGuiModuleCommands::~FImGuiModuleCommands()
|
|
{
|
|
UImGuiSettings::OnSettingsLoaded().RemoveAll(this);
|
|
UnregisterSettingsDelegates();
|
|
}
|
|
|
|
void FImGuiModuleCommands::InitializeSettings()
|
|
{
|
|
RegisterSettingsDelegates();
|
|
|
|
// We manually update key bindings based on ImGui settings rather than using input configuration. This works out
|
|
// of the box in packed and staged builds and it helps to avoid ambiguities where ImGui settings are stored.
|
|
UpdateToggleInputKeyBinding();
|
|
}
|
|
|
|
void FImGuiModuleCommands::RegisterSettingsDelegates()
|
|
{
|
|
if (GImGuiSettings && !GImGuiSettings->OnToggleInputKeyChanged.IsBoundToObject(this))
|
|
{
|
|
GImGuiSettings->OnToggleInputKeyChanged.AddRaw(this, &FImGuiModuleCommands::UpdateToggleInputKeyBinding);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleCommands::UnregisterSettingsDelegates()
|
|
{
|
|
if (GImGuiSettings)
|
|
{
|
|
GImGuiSettings->OnToggleInputKeyChanged.RemoveAll(this);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleCommands::UpdateToggleInputKeyBinding()
|
|
{
|
|
if (GImGuiSettings)
|
|
{
|
|
DebugExecBindings::UpdatePlayerInputs(GImGuiSettings->GetToggleInputKey(), CommandNames::ToggleInput);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleCommands::ToggleInput()
|
|
{
|
|
FImGuiModuleProperties::Get().ToggleInput(ECVF_SetByConsole);
|
|
}
|