mirror of
https://github.com/kevinporetti/UnrealImGui.git
synced 2025-01-19 00:40:32 +00:00
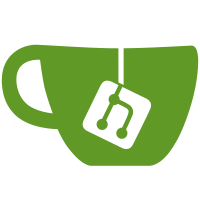
- Moved property variables to ImGui Module Properties. - Moved console command to ImGui Module Commands (one for now but more will be added). - ImGui Module Commands is created by ImGui Module Manager, what means that commands are registered after module is loaded and unregistered when it is unloaded. - Updated settings to allow more convenient use: Added global pointer to default object and event raised when it is loaded.
89 lines
1.9 KiB
C++
89 lines
1.9 KiB
C++
// Distributed under the MIT License (MIT) (see accompanying LICENSE file)
|
|
|
|
#include "ImGuiPrivatePCH.h"
|
|
|
|
#include "ImGuiSettings.h"
|
|
|
|
|
|
UImGuiSettings* GImGuiSettings = nullptr;
|
|
|
|
FSimpleMulticastDelegate& UImGuiSettings::OnSettingsLoaded()
|
|
{
|
|
static FSimpleMulticastDelegate Instance;
|
|
return Instance;
|
|
}
|
|
|
|
|
|
UImGuiSettings::UImGuiSettings()
|
|
{
|
|
#if WITH_EDITOR
|
|
RegisterPropertyChangedDelegate();
|
|
#endif
|
|
}
|
|
|
|
UImGuiSettings::~UImGuiSettings()
|
|
{
|
|
#if WITH_EDITOR
|
|
UnregisterPropertyChangedDelegate();
|
|
#endif
|
|
}
|
|
|
|
void UImGuiSettings::PostInitProperties()
|
|
{
|
|
Super::PostInitProperties();
|
|
|
|
if (IsTemplate())
|
|
{
|
|
GImGuiSettings = this;
|
|
OnSettingsLoaded().Broadcast();
|
|
}
|
|
}
|
|
|
|
void UImGuiSettings::BeginDestroy()
|
|
{
|
|
Super::BeginDestroy();
|
|
|
|
if (GImGuiSettings == this)
|
|
{
|
|
GImGuiSettings = nullptr;
|
|
}
|
|
}
|
|
|
|
#if WITH_EDITOR
|
|
|
|
void UImGuiSettings::RegisterPropertyChangedDelegate()
|
|
{
|
|
if (!FCoreUObjectDelegates::OnObjectPropertyChanged.IsBoundToObject(this))
|
|
{
|
|
FCoreUObjectDelegates::OnObjectPropertyChanged.AddUObject(this, &UImGuiSettings::OnPropertyChanged);
|
|
}
|
|
}
|
|
|
|
void UImGuiSettings::UnregisterPropertyChangedDelegate()
|
|
{
|
|
FCoreUObjectDelegates::OnObjectPropertyChanged.RemoveAll(this);
|
|
}
|
|
|
|
void UImGuiSettings::OnPropertyChanged(class UObject* ObjectBeingModified, struct FPropertyChangedEvent& PropertyChangedEvent)
|
|
{
|
|
if (ObjectBeingModified == this)
|
|
{
|
|
const FName UpdatedPropertyName = PropertyChangedEvent.MemberProperty ? PropertyChangedEvent.MemberProperty->GetFName() : NAME_None;
|
|
|
|
if (UpdatedPropertyName == GET_MEMBER_NAME_CHECKED(UImGuiSettings, ImGuiInputHandlerClass))
|
|
{
|
|
OnImGuiInputHandlerClassChanged.Broadcast();
|
|
}
|
|
else if (UpdatedPropertyName == GET_MEMBER_NAME_CHECKED(UImGuiSettings, SwitchInputModeKey))
|
|
{
|
|
OnSwitchInputModeKeyChanged.Broadcast();
|
|
}
|
|
else if (UpdatedPropertyName == GET_MEMBER_NAME_CHECKED(UImGuiSettings, bUseSoftwareCursor))
|
|
{
|
|
OnSoftwareCursorChanged.Broadcast();
|
|
}
|
|
}
|
|
}
|
|
|
|
#endif // WITH_EDITOR
|