mirror of
https://github.com/kevinporetti/UnrealImGui.git
synced 2025-01-19 00:40:32 +00:00
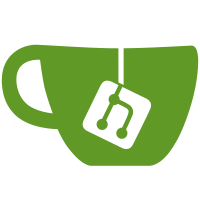
- Added ImGui Input Handler class that allows to customize handling of the keyboard and gamepad input. - Added ImGui Settings to allow specify custom input handler implementation. - Added editor support for ImGui Settings. - Input handling in ImGui Widget is divided for querying the handler (more customizable) and actual input processing based on the handler’s response (fixed and simplified). - Removed a need for checking console state in different input handling functions in ImGui Widget by suppressing keyboard focus support when console is opened.
43 lines
1.1 KiB
C++
43 lines
1.1 KiB
C++
// Distributed under the MIT License (MIT) (see accompanying LICENSE file)
|
|
|
|
#include "ImGuiPrivatePCH.h"
|
|
|
|
#include "ImGuiInputHandler.h"
|
|
|
|
#include "ImGuiContextProxy.h"
|
|
#include "ImGuiModuleManager.h"
|
|
|
|
#include <Engine/Console.h>
|
|
#include <Input/Events.h>
|
|
|
|
|
|
FImGuiInputResponse UImGuiInputHandler::OnKeyDown(const FKeyEvent& KeyEvent)
|
|
{
|
|
// Ignore console open events, so we don't block it from opening.
|
|
if (KeyEvent.GetKey() == EKeys::Tilde)
|
|
{
|
|
return FImGuiInputResponse{ false, false };
|
|
}
|
|
|
|
// Ignore escape event, if they are not meant for ImGui control.
|
|
if (KeyEvent.GetKey() == EKeys::Escape && !HasImGuiActiveItem())
|
|
{
|
|
return FImGuiInputResponse{ false, false };
|
|
}
|
|
|
|
return DefaultResponse();
|
|
}
|
|
|
|
bool UImGuiInputHandler::HasImGuiActiveItem() const
|
|
{
|
|
FImGuiContextProxy* ContextProxy = ModuleManager->GetContextManager().GetContextProxy(ContextIndex);
|
|
return ContextProxy && ContextProxy->HasActiveItem();
|
|
}
|
|
|
|
void UImGuiInputHandler::Initialize(FImGuiModuleManager* InModuleManager, UGameViewportClient* InGameViewport, int32 InContextIndex)
|
|
{
|
|
ModuleManager = InModuleManager;
|
|
GameViewport = InGameViewport;
|
|
ContextIndex = InContextIndex;
|
|
}
|