mirror of
https://github.com/kevinporetti/UnrealImGui.git
synced 2025-01-18 16:30:32 +00:00
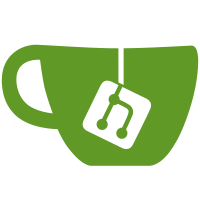
- The old DPI Scale setting was replaced by DPI Scaling Info, which contains information about scale and method of scaling. - ImGui Context Manager handles scaling in ImGui by scaling all styles, rebuilding fonts using a different size, and raising OnFontAtlasBuilt event. - ImGui Module Manager uses OnFontAtlasBuilt event to rebuild font textures. - The update policy in Texture Manager was loosened to update existing resources rather than throwing an exception. This is less strict but it is now more useful since our main texture can now change. The throwing behavior used in the public interface is now handled before calling to the Texture Manager.
194 lines
5.0 KiB
C++
194 lines
5.0 KiB
C++
// Distributed under the MIT License (MIT) (see accompanying LICENSE file)
|
|
|
|
#include "ImGuiPrivatePCH.h"
|
|
|
|
#include "ImGuiModuleSettings.h"
|
|
|
|
#include "ImGuiModuleCommands.h"
|
|
#include "ImGuiModuleProperties.h"
|
|
|
|
|
|
//====================================================================================================
|
|
// UImGuiSettings
|
|
//====================================================================================================
|
|
|
|
UImGuiSettings* UImGuiSettings::DefaultInstance = nullptr;
|
|
|
|
FSimpleMulticastDelegate UImGuiSettings::OnSettingsLoaded;
|
|
|
|
void UImGuiSettings::PostInitProperties()
|
|
{
|
|
Super::PostInitProperties();
|
|
|
|
if (SwitchInputModeKey_DEPRECATED.Key.IsValid() && !ToggleInput.Key.IsValid())
|
|
{
|
|
const FString ConfigFileName = GetDefaultConfigFilename();
|
|
|
|
// Move value to the new property.
|
|
ToggleInput = MoveTemp(SwitchInputModeKey_DEPRECATED);
|
|
|
|
// Remove from configuration file entry for obsolete property.
|
|
if (FConfigFile* ConfigFile = GConfig->Find(ConfigFileName, false))
|
|
{
|
|
if (FConfigSection* Section = ConfigFile->Find(TEXT("/Script/ImGui.ImGuiSettings")))
|
|
{
|
|
if (Section->Remove(TEXT("SwitchInputModeKey")))
|
|
{
|
|
ConfigFile->Dirty = true;
|
|
GConfig->Flush(false, ConfigFileName);
|
|
}
|
|
}
|
|
}
|
|
|
|
// Add to configuration file entry for new property.
|
|
UpdateSinglePropertyInConfigFile(
|
|
UImGuiSettings::StaticClass()->FindPropertyByName(GET_MEMBER_NAME_CHECKED(UImGuiSettings, ToggleInput)),
|
|
ConfigFileName);
|
|
}
|
|
|
|
if (IsTemplate())
|
|
{
|
|
DefaultInstance = this;
|
|
OnSettingsLoaded.Broadcast();
|
|
}
|
|
}
|
|
|
|
void UImGuiSettings::BeginDestroy()
|
|
{
|
|
Super::BeginDestroy();
|
|
|
|
if (DefaultInstance == this)
|
|
{
|
|
DefaultInstance = nullptr;
|
|
}
|
|
}
|
|
|
|
//====================================================================================================
|
|
// FImGuiModuleSettings
|
|
//====================================================================================================
|
|
|
|
FImGuiModuleSettings::FImGuiModuleSettings(FImGuiModuleProperties& InProperties, FImGuiModuleCommands& InCommands)
|
|
: Properties(InProperties)
|
|
, Commands(InCommands)
|
|
{
|
|
#if WITH_EDITOR
|
|
FCoreUObjectDelegates::OnObjectPropertyChanged.AddRaw(this, &FImGuiModuleSettings::OnPropertyChanged);
|
|
#endif
|
|
|
|
// Delegate initializer to support settings loaded after this object creation (in stand-alone builds) and potential
|
|
// reloading of settings.
|
|
UImGuiSettings::OnSettingsLoaded.AddRaw(this, &FImGuiModuleSettings::UpdateSettings);
|
|
|
|
// Call initializer to support settings already loaded (editor).
|
|
UpdateSettings();
|
|
}
|
|
|
|
FImGuiModuleSettings::~FImGuiModuleSettings()
|
|
{
|
|
|
|
UImGuiSettings::OnSettingsLoaded.RemoveAll(this);
|
|
|
|
#if WITH_EDITOR
|
|
FCoreUObjectDelegates::OnObjectPropertyChanged.RemoveAll(this);
|
|
#endif
|
|
}
|
|
|
|
void FImGuiModuleSettings::UpdateSettings()
|
|
{
|
|
if (UImGuiSettings* SettingsObject = UImGuiSettings::Get())
|
|
{
|
|
SetImGuiInputHandlerClass(SettingsObject->ImGuiInputHandlerClass);
|
|
SetShareKeyboardInput(SettingsObject->bShareKeyboardInput);
|
|
SetShareGamepadInput(SettingsObject->bShareGamepadInput);
|
|
SetShareMouseInput(SettingsObject->bShareMouseInput);
|
|
SetUseSoftwareCursor(SettingsObject->bUseSoftwareCursor);
|
|
SetToggleInputKey(SettingsObject->ToggleInput);
|
|
SetCanvasSizeInfo(SettingsObject->CanvasSize);
|
|
SetDPIScaleInfo(SettingsObject->DPIScale);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetImGuiInputHandlerClass(const FStringClassReference& ClassReference)
|
|
{
|
|
if (ImGuiInputHandlerClass != ClassReference)
|
|
{
|
|
ImGuiInputHandlerClass = ClassReference;
|
|
OnImGuiInputHandlerClassChanged.Broadcast(ClassReference);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetShareKeyboardInput(bool bShare)
|
|
{
|
|
if (bShareKeyboardInput != bShare)
|
|
{
|
|
bShareKeyboardInput = bShare;
|
|
Properties.SetKeyboardInputShared(bShare);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetShareGamepadInput(bool bShare)
|
|
{
|
|
if (bShareGamepadInput != bShare)
|
|
{
|
|
bShareGamepadInput = bShare;
|
|
Properties.SetGamepadInputShared(bShare);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetShareMouseInput(bool bShare)
|
|
{
|
|
if (bShareMouseInput != bShare)
|
|
{
|
|
bShareMouseInput = bShare;
|
|
Properties.SetMouseInputShared(bShare);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetUseSoftwareCursor(bool bUse)
|
|
{
|
|
if (bUseSoftwareCursor != bUse)
|
|
{
|
|
bUseSoftwareCursor = bUse;
|
|
OnUseSoftwareCursorChanged.Broadcast(bUse);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetToggleInputKey(const FImGuiKeyInfo& KeyInfo)
|
|
{
|
|
if (ToggleInputKey != KeyInfo)
|
|
{
|
|
ToggleInputKey = KeyInfo;
|
|
Commands.SetKeyBinding(FImGuiModuleCommands::ToggleInput, ToggleInputKey);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetCanvasSizeInfo(const FImGuiCanvasSizeInfo& CanvasSizeInfo)
|
|
{
|
|
if (CanvasSize != CanvasSizeInfo)
|
|
{
|
|
CanvasSize = CanvasSizeInfo;
|
|
OnCanvasSizeChangedDelegate.Broadcast(CanvasSize);
|
|
}
|
|
}
|
|
|
|
void FImGuiModuleSettings::SetDPIScaleInfo(const FImGuiDPIScaleInfo& ScaleInfo)
|
|
{
|
|
if (DPIScale != ScaleInfo)
|
|
{
|
|
DPIScale = ScaleInfo;
|
|
OnDPIScaleChangedDelegate.Broadcast(DPIScale);
|
|
}
|
|
}
|
|
|
|
#if WITH_EDITOR
|
|
|
|
void FImGuiModuleSettings::OnPropertyChanged(class UObject* ObjectBeingModified, struct FPropertyChangedEvent& PropertyChangedEvent)
|
|
{
|
|
if (ObjectBeingModified == UImGuiSettings::Get())
|
|
{
|
|
UpdateSettings();
|
|
}
|
|
}
|
|
|
|
#endif // WITH_EDITOR
|