mirror of
https://github.com/kevinporetti/UnrealImGui.git
synced 2025-07-03 03:30:33 +00:00
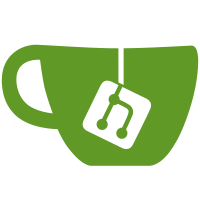
- Divided ImGui settings into UImGuiSettings persistent object and FImGuiModuleSettings proxy that provides interface for other classes and handles delayed loading of UImGuiSettings. - Removed from FImGuiModuleProperties and FImGuiModuleCommands direct dependencies on UImGuiSettings. - Simplified FImGuiModuleProperties making it more robust when moving data after hot-reloading. - Inverted binding logic by injecting FImGuiModuleProperties and FImGuiModuleCommands into FImGuiModuleSettings and letting it take care of synchronization. Dependencies are setup by module manager. - Added to module manager FImGuiModuleSettings and interface to access it. - Cleaned interface of FImGuiInputHandlerFactory and removed direct dependency on settings.
49 lines
1.2 KiB
C++
49 lines
1.2 KiB
C++
// Distributed under the MIT License (MIT) (see accompanying LICENSE file)
|
|
|
|
#include "ImGuiPrivatePCH.h"
|
|
|
|
#include "ImGuiInputHandlerFactory.h"
|
|
|
|
#include "ImGuiInputHandler.h"
|
|
|
|
|
|
UImGuiInputHandler* FImGuiInputHandlerFactory::NewHandler(const FStringClassReference& HandlerClassReference, FImGuiModuleManager* ModuleManager, UGameViewportClient* GameViewport, int32 ContextIndex)
|
|
{
|
|
UClass* HandlerClass = nullptr;
|
|
if (HandlerClassReference.IsValid())
|
|
{
|
|
HandlerClass = HandlerClassReference.TryLoadClass<UImGuiInputHandler>();
|
|
|
|
if (!HandlerClass)
|
|
{
|
|
UE_LOG(LogImGuiInputHandler, Error, TEXT("Couldn't load ImGui Input Handler class '%s'."), *HandlerClassReference.ToString());
|
|
}
|
|
}
|
|
|
|
if (!HandlerClass)
|
|
{
|
|
HandlerClass = UImGuiInputHandler::StaticClass();
|
|
}
|
|
|
|
UImGuiInputHandler* Handler = NewObject<UImGuiInputHandler>(GameViewport, HandlerClass);
|
|
if (Handler)
|
|
{
|
|
Handler->Initialize(ModuleManager, GameViewport, ContextIndex);
|
|
Handler->AddToRoot();
|
|
}
|
|
else
|
|
{
|
|
UE_LOG(LogImGuiInputHandler, Error, TEXT("Failed attempt to create Input Handler: HandlerClass = %s."), *GetNameSafe(HandlerClass));
|
|
}
|
|
|
|
return Handler;
|
|
}
|
|
|
|
void FImGuiInputHandlerFactory::ReleaseHandler(UImGuiInputHandler* Handler)
|
|
{
|
|
if (Handler)
|
|
{
|
|
Handler->RemoveFromRoot();
|
|
}
|
|
}
|